My client, a major hospital chain in Miami, wanted to add a Transfer action on PA52 – Individual Action when an internal applicant’s acceptance was keyed on PA45 – Requisition Offers:
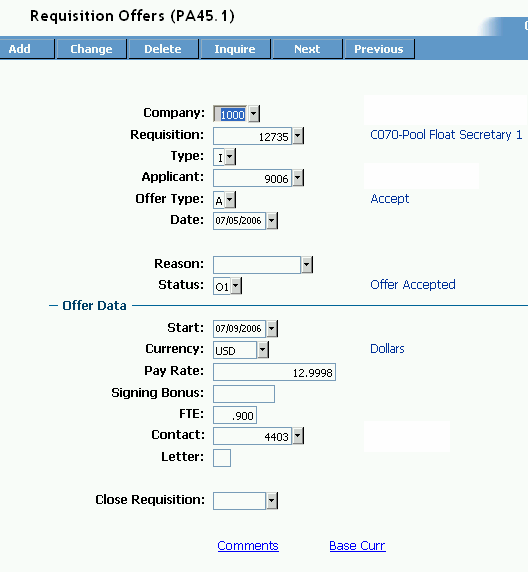
By using Design Studio we were able to automate this process; however we did face some interesting challenges during our design.
Conditional event based “trigger”
The triggering of our JavaScript function would be conditional upon the “Add” or “Change” buttons being pressed. We elected to use the Form object OnBeforeTransaction event available in the Script section of Design Studio. Our trigger was based upon the function code (fc) being either “C” (Change) or “A” (Add) – meaning the Change or Add button had been pressed.

The next conditions to check were whether the applicant Type was “Internal” and whether the Offer Type was “Accept.”
The Type field is named “select1” and the Offer Type field is named “select2.”
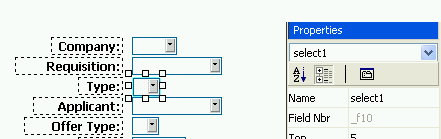
We got the values of these fields and assigned them to variables (which we also named select1 and select2). We then checked these variables to make sure the required conditions are met. [JavaScript uses the double equal sign = = when comparing values and the single equal sign = when assigning values.]
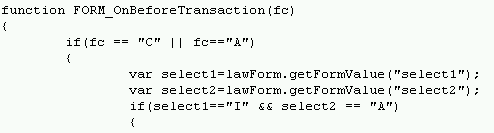
Note that unlike the value of fc, which could be either “C” or “A”, both values for select1 and select2 had to be met. [In JavaScript, the double ampersand && equals “and” and the double pipe || equals “or.”]
Since we’ve now validated that our form and field value conditions have been met, we’re ready to move forward.
Calling functions to perform tasks
Since we’re going to perform several different tasks we decided to keep them separate and built a different function for each. The code below demonstrates how we’re calling each function in the order we want them processed.
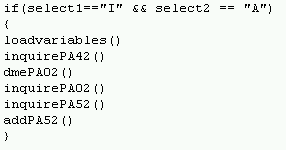
Variables
My JavaScript for Dummies book tells me that you can’t define a variable within a function and have it available outside of that function. I found this to be true when I defined my variables within my inquirePA42() function but couldn’t use them in later functions.
I remembered seeing within Lawson’s ProcessFlow requisition approval JavaScript sample (recsum.htm) that they defined their variables using a loadvariables() function so copied that methodology.
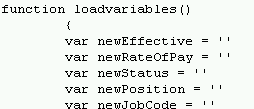
Gathering required information
In order to eventually add the Transfer action on PA52, we need a lot more data than is available on our PA45 form. This requires that we get information from the job Requisition (PA42), the Position (PA02) and the employee’s current values from PA52.
We decided to inquire on the actual forms (AGS) rather than the data tables (DME) because it was easier to reference the data we needed and because we only needed one record instead of multiple records.
The first form we needed additional data from was the PA42 – Requisition form. We needed to pass selection criteria in order to inquire on the correct record. We were able to hard code the company but needed to use the Requisition number (stored in the “text2” form field).
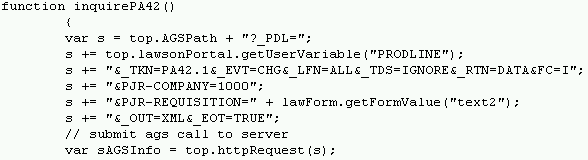
We defined the “s” variable with the AGS call and then submitted the AGS call through the sAGSInfo variable definition. We could now reference the AGS object and get the field values to assign to our variables. (Be sure to include the _RTN=DATA portion in the AGS call or you won’t be able to reference the values you need to assign to your variables.)

In order to perform the AGS call on the Position (PA02) we needed the Position value assigned to the variable newPosition but we also needed the position’s effective date. To get this date, we did perform a DME call against the data table but then used the inquire AGS call against PA02 (again, because it was easier to reference the data values).
The last inquire AGS call we performed was against the PA52 form and this was done in order to quickly access the employee’s current values. When you add the information to PA52 you can’t key the same data into the Selected Items “Change To” field if it is equal to the “Current Value.”
Since we had all of the data values we need to “change to” from the PA45, PA42 and PA02 forms and have them assigned to specific variables, we needed to compare them to their current values on PA52. If the “change to” value was equal to the “current value” we updated the variable to a null value.
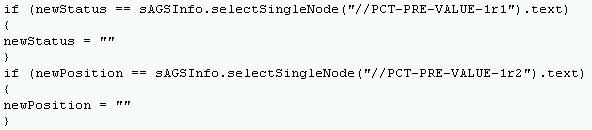
[Note the inquire AGS call being set to _OUT=XML you need reference the fields as they are returned in XML. We used the API Builder to get the correct formatting.]
Adding the PA52 Transfer Action
Having the data (and updating it as needed) we were ready to perform our last function - we were ready to add the transfer action via an AGS call to PA52.
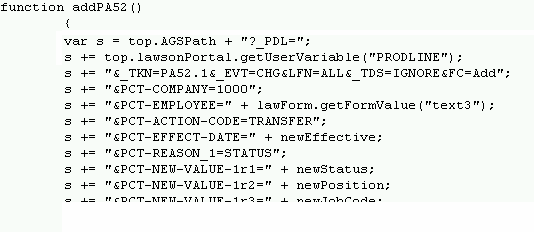
We were actually told we couldn’t add the transaction on PA52 because of the form’s functionality. This form requires you to add the Company, Employee, and Action and then Inquire before you can fill in the remaining fields and add the transaction.
We got around this by using _EVT=CHG within the AGS call instead of _EVT=ADD as you would normally expect. Since FC=Add was set in the AGS call the system added the transaction.
In some cases, the add function to PA52 would fail – this occurred during our testing when the supervisor data was missing on PA02 or when the new pay was outside of the position’s range. In order to let the user know that the PA52 was added correctly we included the system message in an alert box.

|